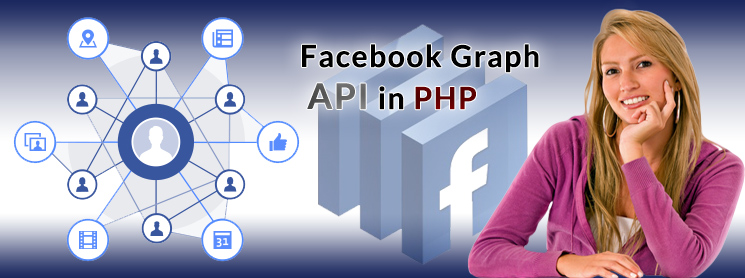
Hi, I am Ramanjineyulu. I, along with my colleague Abhinish Kumar, worked on developing Facebook application using facebook Graph API (in PHP) recently. This Application helps retrieve the all public details of a user and displays it in a useful format. We found this very interesting and want to share in easy steps how we went about it.
What is Facebook Application?
Facebook Applications are developed to enhance one’s experience on Facebook. It is provided by the social networking giant Facebook for third-party developers to create their own applications and services that access Facebook data.
Facebook provides SDK for development of Facebook Applications in various platforms like PHP, iOS etc. Facebook SDK for PHP is provided by Facebook for Application development in PHP. Facebook keeps updating its SDKs and it is recommended to use the latest one.
Graph API
The Graph API is the core of Facebook Platform, enabling developers to read from and write data into Facebook. The Graph API presents a simple, consistent view of the Facebook social graph, uniformly representing objects in the graph (e.g., people, photos, events, and pages) and the connections between them (e.g., friend relationships, shared content, and photo tags).
Getting Started
After logging in to Facebook with your valid credentials, you need to create your own application to enhance your experience on Facebook. For this you need to follow the following steps:
Go to developer page, here you can choose Apps tab and then click on ‘Create new App’. After that follow the instructions and give the valid name and namespace for that App. Here are the sub-steps:
• Visit Facebook’s Developer Site (you need a Facebook verified account to create the application)
• In the ‘docs’ section you can find various SDKs, download the SDK for PHP. You will get the SDK as a zip file, extract it to any location. In the SRC folder, you will get a Facebook folder containing the library files; copy the entire Facebook folder into the root of your application.
• Include the various files you need for your application
• Create an application on Facebook and note your App ID and App Secret, you will need them while creating your application, provide other details as necessary.
• Go to settings and provide the settings you need for your app. You are now ready to start developing your Facebook Application.
Developing the Application
First you need to configure all those files which are required to develop the Application. Next you can follow these steps:
• You will need to have configured a Facebook App, which you can obtain from the App Dashboard.
• Now initialize the SDK with your app ID and secret:
FacebookSession::setDefaultApplication(‘YOUR_APP_ID’,’YOUR_APP_SECRET’);
• The SDK can be used to support login to your site using a Facebook account. On the server-side, the SDK provides helper classes for the most common scenarios.
• For your website you can use the FacebookRedirectLoginHelper. Generate the login URL to redirect visitors to with the getLoginUrl(); method, redirect them, and then process the response from Facebook with the getSessionFromRedirect(); method, which returns a FacebookSession.
$helper= new FacebookRedirectLoginHelper(‘your redirect Url’);
$loginUrl= $helper->getLoginUrl();
• Now you have to set the URL for getting response to your Facebook Application. Remember, we already created this earlier. Get this by going to your application -> Settings -> Advanced tab-> valid OAuth Redirect URls -> type your redirect url here.
• Once you have a FacebookSession you can begin making calls to the Graph API with FacebookRequest.
$request=(newFacebookRequest ($session, ‘GET’, ‘/me’))->execute()->getGraphObject();
Now you have the $request as a GraphObject. By Accessing it you can get the various details of user.
Sample WebApplication in PHP to Get Details
?php session_start(); /** * Description : This application will helps you to get the all public details of user * first we should include all required files provided by Facebook SDK */ include 'Facebook/FacebookCurlHttpClient.php'; include 'Facebook/FacebookRedirectLoginHelper.php'; require_once( 'Facebook/FacebookRequest.php' ); require_once( 'Facebook/FacebookResponse.php' ); require_once( 'Facebook/FacebookSDKException.php' ); require_once( 'Facebook/FacebookRequestException.php' ); require_once( 'Facebook/FacebookAuthorizationException.php' ); require_once( 'Facebook/GraphObject.php' ); require_once( 'Facebook/GraphUser.php' ); use Facebook\FacebookRequest; use Facebook\FacebookRequestException; include 'Facebook/FacebookSession.php'; Use Facebook\FacebookSession; Use Facebook\FacebookRedirectLoginHelper; /** * here you have to provide your application ID and SECRET * which are given by facebook after creating the application */ FacebookSession::setDefaultApplication('255253844664429', '5b1644e8f084191cb1bbb2d41c7d73f3'); $helper = new FacebookRedirectLoginHelper('http://localhost.testapp.com/fbapp_details.php'); $session = $helper->getSessionFromRedirect(); echo "<title>FBApp-Details</title>"; /** * Code to get the public details of user */ if ($session != NULL) { try { $user_profile = (new FacebookRequest( $session, 'GET', '/me' ))->execute()->getGraphObject(); echo "</br>"; echo "Name : " . $user_profile->getProperty('name') . "</br>"; echo "Email : " . $user_profile->getProperty('email') . "</br>"; echo "Gender : " . $user_profile->getProperty('gender') . "</br>"; echo "Bio : " . $user_profile->getProperty('bio') . "</br>"; echo "Date of Birth : " . $user_profile->getProperty('birthday') . "</br>"; } catch (FacebookRequestException $e) { echo "Exception occured, code: " . $e->getCode(); echo " with message: " . $e->getMessage(); } } else { echo '<a href="' . $helper->getLoginUrl(array( 'scope' => 'user_likes,publish_actions,read_friendlists,email,user_birthday,user_location, user_about_me,user_friends,user_photos')) . '"><font size="6"><i><strong> Get Details</strong></i></font></a>'; } ?>
So you see how interesting it is to develop a web application that interacts with Facebook and gets you the public details of the user.
Similarly, we can develop web applications like post a story on user’s wall, upload photos, tag pics with his friends list, get the user liked pages etc. by using Facebook Graph API in PHP.
Hope you found this interesting too. Keep watching the space for more!